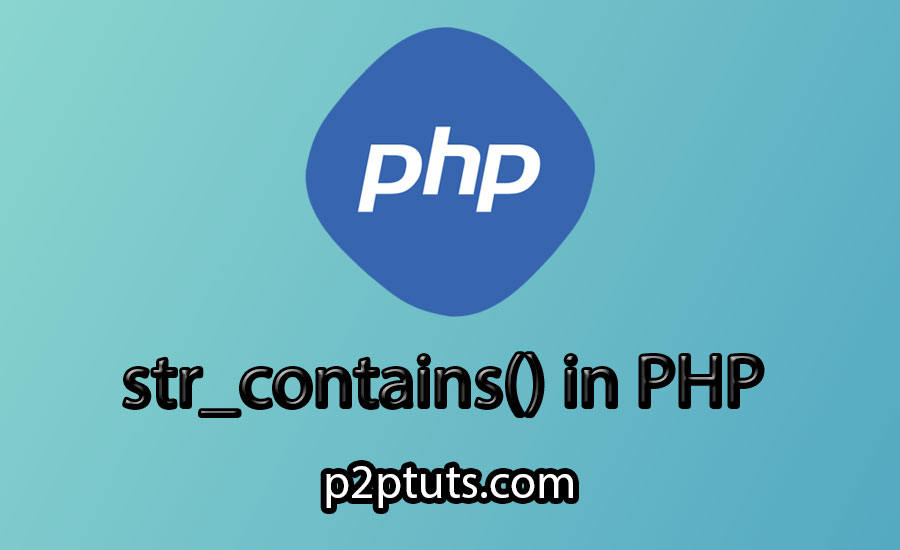
str_contains() Function in PHP - Checking Substrings
- by Admin
- 196 Views
- PHP
- Codeigniter
- Laravel
Overview of str_contains() in PHP
In PHP, the str_contains
function is part of version 8.0 and later, providing the ability to check whether a string contains a substring or not. This is a crucial feature that simplifies string processing, reducing complexity and enhancing performance.
Syntax of str_contains
The syntax of str_contains
is straightforward:
bool str_contains ( string $haystack , string $needle )
Where:
$haystack
: The string containing the data to be checked.$needle
: The substring to check for in$haystack
.
Example:
$string = "Hello, World!";
$containsWorld = str_contains($string, "World"); // Result: true
Usage of str_contains with detailed examples
1. Checking the presence of a keyword in text
$text = "PHP is a powerful scripting language.";
$keyword = "powerful";
$containsKeyword = str_contains($text, $keyword); // Result: true
2. Determining the appearance of a substring in a file path
$filePath = "/var/www/html/project/files/document.txt";
$extension = ".txt";
$containsExtension = str_contains($filePath, $extension); // Result: true
PHP str_contains multiple
When checking multiple substrings simultaneously, str_contains
supports the verification of multiple substrings within a main string.
$text = "The quick brown fox jumps over the lazy dog";
$keywords = ["fox", "dog"];
$containsKeywords = array_map(fn($keyword) => str_contains($text, $keyword), $keywords);
// Result: [true, true]
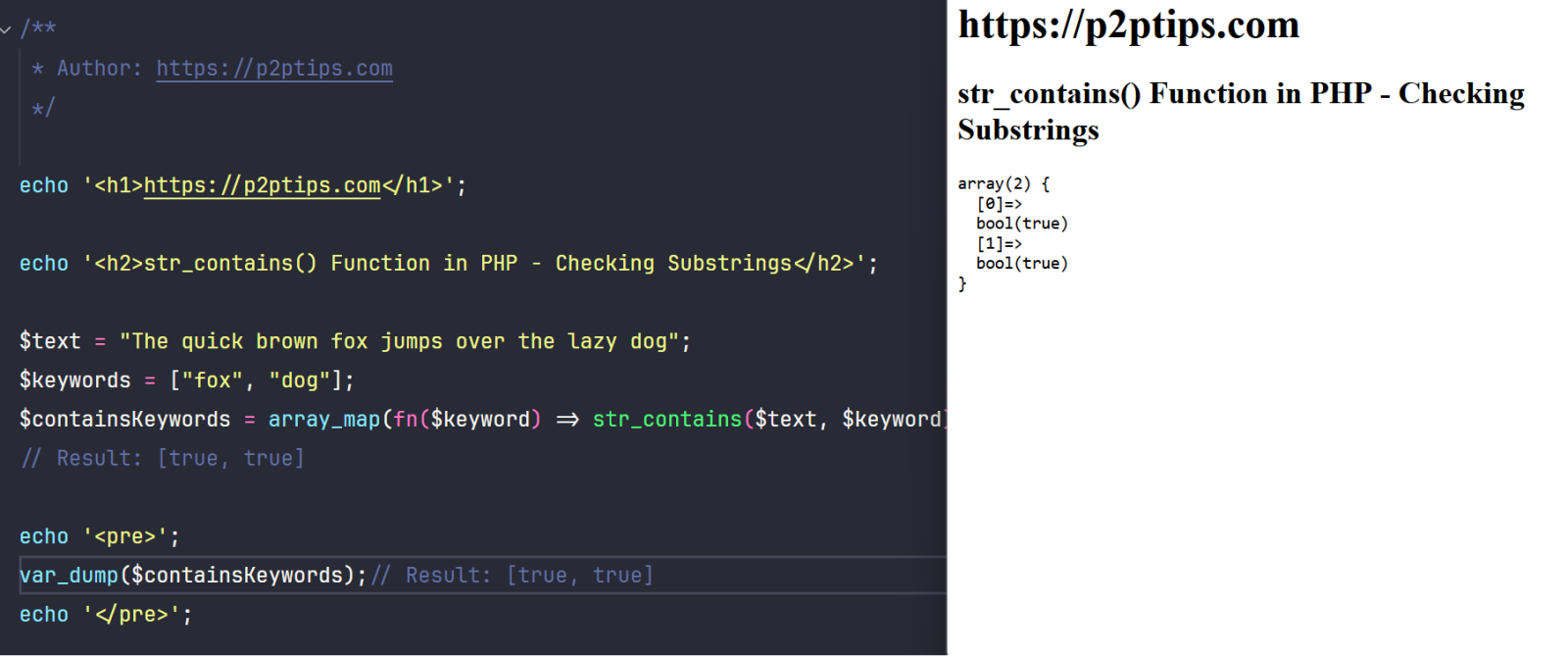
Comparison of str_contains with other string search functions in PHP
When compared to other string search functions like strpos
, stripos
, and preg_match
, str_contains
has advantages in terms of simplicity and readability in many cases.
Example with strpos:
$text = "Hello, World!";
$containsWorld = strpos($text, "World") !== false; // Result: true
Alternative options to str_contains: strpos(), stripos(), preg_match()
If your PHP version does not support str_contains
or you are working with older versions, alternative functions like strpos
, stripos
, or preg_match
can be used.
Example with stripos
as a replacement for str_contains
:
$text = "Hello, World!";
$containsWorld = stripos($text, "World") !== false; // Result: true
Performance of str_contains compared to other string search functions
str_contains
generally exhibits better performance compared to traditional string search functions like strpos
and preg_match
, especially when checking for the existence of a substring in a long string.
Conclusion
str_contains
is a significant feature in PHP, streamlining string checking processes and improving performance. In many scenarios, it can replace traditional string search functions, providing readable and efficient code. However, if working with older PHP versions, consider alternative string search functions.
Keywords:
- Str_contains PHP w3schools
- PHP strpos
- call to undefined function str_contains()
- php str_contains multiple
- Str_contains w3
- Strpos
- Str_contains array php
- Check string contains array php