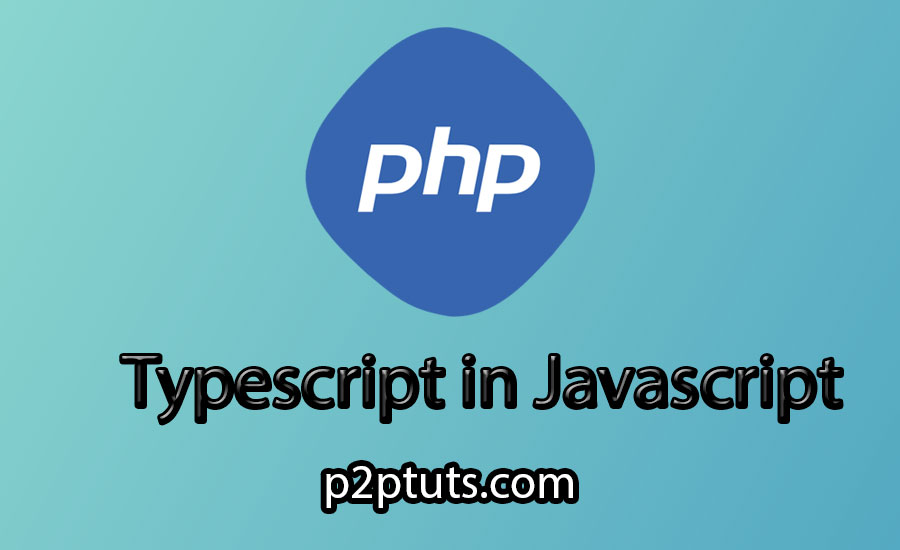
Comprehensive Guide to TypeScript: Exploring Features and Benefits
- by Admin
- 221 Views
- Javascript
1. What is TypeScript?
TypeScript is an open-source programming language, an extension of JavaScript, created for developing large and maintainable applications. TypeScript introduces static typing, enhancing code clarity and reliability.
Example:
function greet(name: string): string {
return `Hello, ${name}!`;
}
let message: string = greet("TypeScript");
console.log(message);
2. Features and Benefits of TypeScript
- Static Data Types: TypeScript supports static data types, aiding in error detection and performance improvement.
- Object-Oriented Programming: It supports object-oriented programming features such as classes and interfaces.
- Performance and Maintenance: TypeScript facilitates the development of easily maintainable and high-performance source code.
3. TypeScript vs. JavaScript
TypeScript is a superset of JavaScript, adding object-oriented programming features and static data types.
Example:
// JavaScript
function greet(name) {
return "Hello, " + name + "!";
}
// TypeScript
function greet(name: string): string {
return `Hello, ${name}!`;
}
4. Installing and Setting Up TypeScript
To install TypeScript, use npm (Node Package Manager) and then set up the development environment with the tsconfig.json configuration file.
Example:
# Install TypeScript globally
npm install -g typescript
# Initialize TypeScript configuration file
tsc --init
5. Data Types in TypeScript
TypeScript supports various data types such as number, string, boolean, arrays, tuples, and any.
Example:
let age: number = 25;
let name: string = "John";
let isStudent: boolean = true;
let scores: number[] = [90, 85, 92];
let student: [string, number] = ["Alice", 22];
6. TypeScript Type System
The TypeScript type system helps control data types and ensures program accuracy.
Example:
let numberVar: number = 10;
numberVar = "Hello"; // Compilation error: Incompatible data type
7. Variables and Constants in TypeScript
Use let
to declare variables and const
for constants.
Example:
let count: number = 5;
const PI: number = 3.14;
8. Scope and Encapsulation in TypeScript
TypeScript supports variable scope and encapsulation through code blocks and declarations.
Example:
function scopeExample() {
if (true) {
let localVar: string = "Local Variable";
console.log(localVar);
}
console.log(localVar); // Compilation error: Cannot access local variable outside the code block.
}
9. Arrow Function and Lambda Expression in TypeScript
Use arrow functions and lambda expressions for concise and readable code.
Example:
// Arrow Function
let add = (a: number, b: number) => a + b;
// Lambda Expression
let multiply = (x: number) => x * 2;
10. Useful Libraries and Tools in TypeScript
TypeScript has many useful libraries and tools such as Axios, Lodash, and ESLint to optimize the development process.
Example:
# Install Axios
npm install axios
In summary, TypeScript is not just an extension of JavaScript; it brings powerful features and benefits, enhancing the strength and flexibility for modern software projects.