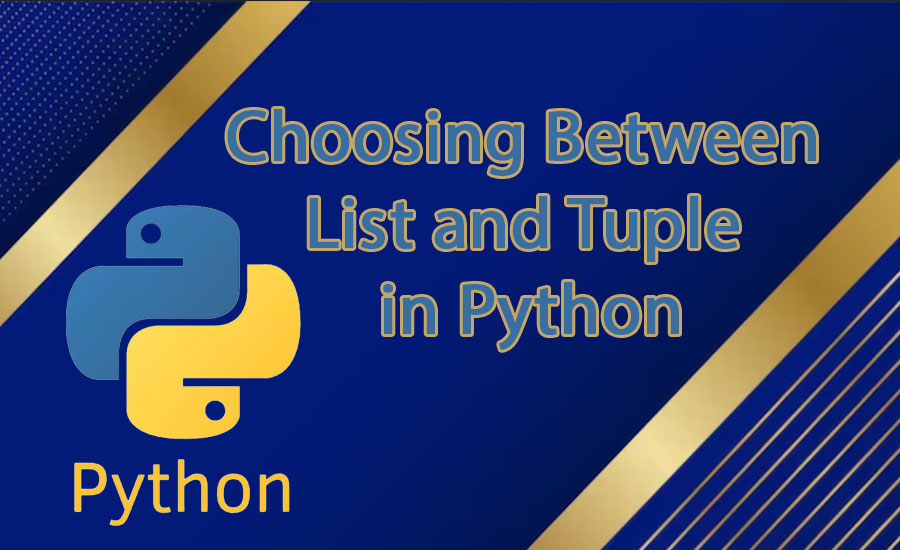
Difference between list and tuple in python with examples
Introduction
In Python, lists and tuples are two very important types of data structures. Each type has its own characteristics and uses. This article will difference between list and tuple in python with examples, helping you better understand each type and use them effectively. Python, renowned for its versatility and simplicity, offers two fundamental data structures for storing collections of elements: lists and tuples. While both may seem interchangeable, they serve distinct purposes in programming. This guide elucidates the differences between lists and tuples in Python, accompanied by practical examples to solidify comprehension.
Mutability
- Lists: Mutable, allowing dynamic modifications such as appending, removing, or modifying elements.
- Tuples: Immutable, once created, elements cannot be changed, added, or removed.
Syntax
- Lists: Enclosed in square brackets
[]
. - Tuples: Enclosed within parentheses
()
.
Usage
- Lists: Ideal for scenarios requiring dynamic data manipulation, such as managing user inputs, tracking inventory, or logging data.
- Tuples: Suited for situations where data integrity and immutability are paramount, such as storing coordinates or configuration settings.
Example
# List Example: Managing a To-Do List
todo_list = ["Complete assignment", "Buy groceries", "Attend meeting"]
todo_list.append("Exercise") # Add a new task
print(todo_list) # Output: ['Complete assignment', 'Buy groceries', 'Attend meeting', 'Exercise']
# Tuple Example: Storing Coordinates
coordinate = (10, 20)
print("Initial Coordinate:", coordinate)
# Attempting to modify a tuple element
# coordinate[0] = 15 # Error! Tuples are immutable
# print("Modified Coordinate:", coordinate)
In the above example, you can see how a new element can be added to the list but cannot be added to the set because the set is immutable.
Comparison
List | Tuple | |
---|---|---|
Mutability | Can be changed | Cannot be changed |
Syntax | Square brackets [] |
Parentheses () |
Speed | Often slower | Often faster |
Memory | Uses more memory | Uses less memory |
Usage | When data needs to | When data needs to |
be changed | be consistent |
Summary
In short, lists and sets in Python are two widely used data types to store collections of elements. While lists are mutable (element can be added, removed, replaced) and can contain many different types of elements, tuples are immutable and can only contain non-duplicate elements, no in order. The choice of using lists or sets depends on the specific requirements of the program, in which lists are more suitable for cases where it is necessary to manipulate ordered and potentially changing data, while sets More suitable for cases where data needs to be stored without duplicates, without order, and requires quick access to elements.
Choosing Between List and Tuple in Python