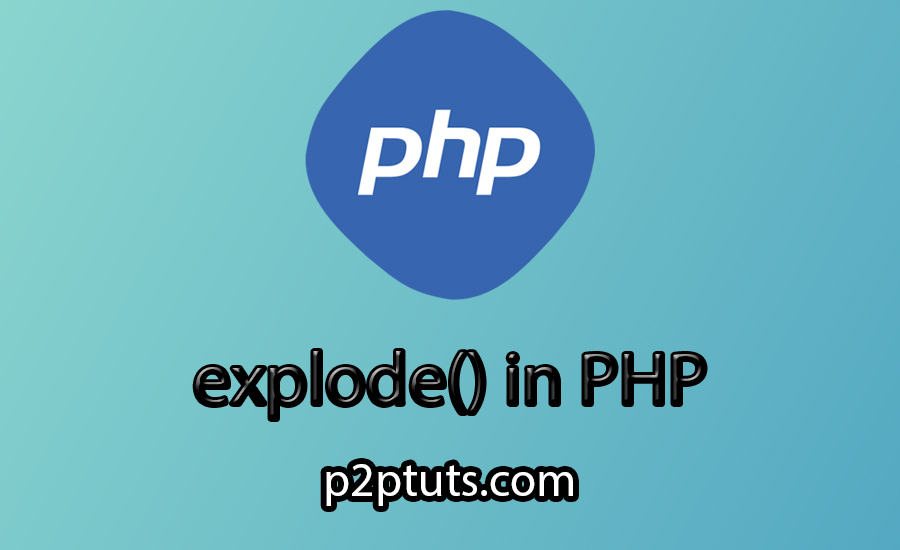
Instructions for using explode() function in php
Overview
The explode()
function in PHP is a function used to split a string into an array of strings based on a specified delimiter. This function returns an array containing strings generated by splitting the original string at the boundaries formed by the delimiter.
The explode()
function can be beneficial for handling and manipulating data from user input, files, etc. For example, when working with comma-separated values (CSV), processing log files, or parsing command-line arguments.
Syntax and Parameters
The syntax of the explode()
function in PHP is as follows:
explode(string $separator, string $string, int $limit = PHP_INT_MAX) : array
Where:
$separator
is a required delimiter string. Theexplode()
function will split the$string
at each occurrence of this delimiter. If the delimiter string is an empty string (""), theexplode()
function will throw aValueError
.$string
is the required input string. Theexplode()
function will split this string into an array of strings.$limit
is an optional parameter that determines the maximum number of elements in the returned array. Possible values include:- Greater than 0: The returned array will contain a maximum of
$limit
elements, with the last element containing the remaining portion of the$string
. - Less than 0: The returned array will contain all elements except the last
$limit
elements. - Equal to 0: The returned array will contain a single element, which is the entire
$string
.
- Greater than 0: The returned array will contain a maximum of
Return Values
The explode()
function returns an array of strings generated by splitting the $string
at the boundaries formed by the $separator
string.
If the $separator
string does not appear in the $string
, the returned array will contain a single element, which is the entire $string
.
If the $separator
string appears at the beginning or end of the $string
, the returned array will have an empty element at the corresponding first or last position.
Examples of Using the Function
Example 1: Splitting a String into an Array
<?php
$str = "Hello world. It's a beautiful day.";
$arr = explode(" ", $str);
print_r($arr);
?>
Output:
Array
(
[0] => Hello
[1] => world.
[2] => It's
[3] => a
[4] => beautiful
[5] => day.
)
In this example, the explode()
function splits the $str
string into an array of strings based on the space (" ") delimiter.
Example 2: Using the $limit
Parameter to Limit the Number of Elements in the Returned Array
<?php
$str = "one,two,three,four";
// zero limit
print_r(explode(",", $str, 0));
// positive limit
print_r(explode(",", $str, 2));
// negative limit
print_r(explode(",", $str, -1));
?>
Output:
Array
(
[0] => one,two,three,four
)
Array
(
[0] => one
[1] => two,three,four
)
Array
(
[0] => one
[1] => two
[2] => three
)
In this example, the explode()
function splits the $str
string into an array of strings based on the comma (",") delimiter. Depending on the value of the $limit
parameter, the returned array will have different numbers of elements.
Considerations When Using the Function
- The
explode()
function is safe for binary data, meaning it can handle strings containing special or non-displayable characters. - The
explode()
function can only split a string based on a fixed delimiter and does not support regular expressions. If splitting based on a regular expression is needed, thepreg_split()
function can be used. - The
explode()
function requires the$separator
parameter to come before the$string
parameter. The order of these two parameters cannot be reversed like theimplode()
function.
Common Use Cases
Handling CSV Data
CSV is a common data format where values are separated by commas (or another character). The explode()
function can be used to split a CSV data row into an array of values. For example:
<?php
// A CSV data row
$csv = "John,Doe,35,Male,New York";
// Split the data row into an array of values
$values = explode(",", $csv);
// Print the values
echo "Name: " . $values[0] . " " . $values[1] . "\n";
echo "Age: " . $values[2] . "\n";
echo "Gender: " . $values[3] . "\n";
echo "City: " . $values[4] . "\n";
?>
Output:
Name: John Doe
Age: 35
Gender: Male
City: New York
Comparing explode()
with Similar Functions in PHP
In PHP, there are other functions with similar purposes, but with important differences. Below are some similar functions and how they compare to explode()
:
str_split()
: This function splits a string into an array of characters. Its input parameters include the string to split and the maximum length of each array element. Unlikeexplode()
, it does not use a delimiter string but an integer to determine the length of each array element. For example:
<?php
$str = "Hello";
// Split the string into an array of characters
print_r(str_split($str));
// Split the string into an array of strings with a length of 2 characters each
print_r(str_split($str, 2));
?>
Output:
Array
(
[0] => H
[1] => e
[2] => l
[3] => l
[4] => o
)
Array
(
[0] => He
[1] => ll
[2] => o
)
preg_split()
: This function splits a string into an array based on a regular expression. Its input parameters include the regular expression, the string to split, the limit on the number of elements in the array, and optional flags. It differs fromexplode()
in that it can split a string using multiple delimiters or more complex patterns. For example:
<?php
$str = "red,green;blue|yellow";
// Split the string using , ; |
print_r(preg_split("/[,;|]/", $str));
// Split the string using characters in the range a-z
print_r(preg_split("/[a-z]+/", $str));
?>
Output:
Array
(
[0] => red
[1] => green
[2] => blue
[3] => yellow
)
Array
(
[0] =>
[1] => ,
[2] => ;
[3] => |
[4] =>
)
Tips and Tricks When Using explode()
- To split a string into an array of words, the
str_word_count()
function can be used instead ofexplode()
.str_word_count()
can determine words in a string more accurately, as it can ignore special characters, numbers, or extra whitespace. For example:
<?php
$str = "Hello, world! This is a test.";
// Split the string into an array of words using explode()
print_r(explode(" ", $str));
// Split the string into an array of words using str_word_count()
print_r(str_word_count($str, 1));
?>
Output:
Array
(
[0] => Hello,
[1] => world!
[2] => This
[3] => is
[4] => a
[5] => test.
)
Array
(
[0] => Hello
[1] => world
[2] => This
[3] => is
[4] => a
[5] => test
)
- To split a string into an array of sentences, the
preg_split()
function with a regular expression to identify sentence-ending punctuation marks can be used. For example:
Output:
Array
(
[0] => Hello
[1] => How are you
[2] => I'm fine, thank you
)
Conclusion
The explode()
function in PHP is a useful tool for splitting a string into an array of strings based on a specified delimiter. It can be employed to handle and manipulate data from user input, files, etc. In this article, we introduced the syntax, parameters, return values, examples, considerations, common use cases, similar functions, and tips and tricks when using the explode()
function in PHP. We hope this article helps you understand and utilize the explode()
function more effectively.