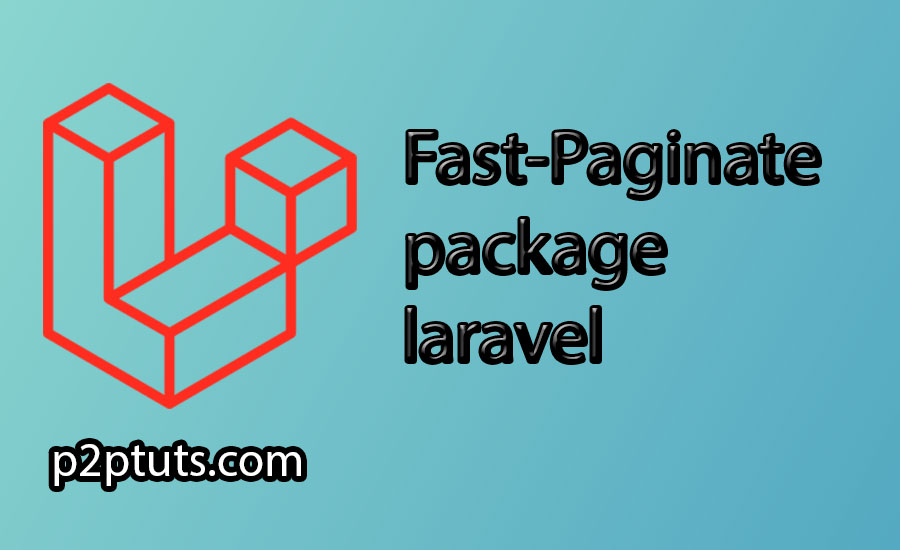
Introduction to Fast-Paginate Package for Laravel
Laravel is a popular PHP framework favored by many developers for its flexibility, ease of use, and support for numerous useful features. One of those features is pagination, allowing you to display data page by page rather than all at once. However, traditional pagination in Laravel may face performance issues, especially when dealing with large datasets and complex queries. So, how can you improve the pagination performance in Laravel? The answer lies in using the Fast-Paginate package.
Introduction to the Fast-Paginate Package
Fast-Paginate is an extension package for Laravel that enables faster pagination by utilizing a SQL method similar to a "deferred join." This technique defers access to the required columns until after applying offset and limit. In this package's case, it doesn't actually perform a join but instead uses a where-in with a subquery. By employing this technique, a subquery is created that can be optimized with specific indexes to achieve the highest speed, and then the result is used to fetch the full rows. The SQL statement looks like this:
SELECT * FROM contacts -- Full data you want to display to the user.
WHERE contacts.id IN ( -- "Deferred join" or subquery, in our case.
SELECT id FROM contacts -- Pagination, accessing the least amount of data - only IDs.
LIMIT 15 OFFSET 150000
)
The benefits of this approach may vary depending on your data, but it allows the database to check the least amount of data necessary to meet user requirements. There is no guarantee that this method will outperform traditional offset/limit pagination, although it might, so you should test it on your data. If you want to delve into the theory of this package.
Using the Fast-Paginate Package
To use the Fast-Paginate package, you need to install it via Composer:
composer require hammerstone/fast-paginate
No additional configuration is needed. The service provider will be automatically loaded by Laravel. Afterward, you can use the fastPaginate()
method instead of the regular paginate()
method. The method signatures are the same, and relationships are also supported:
User::first()->posts()->fastPaginate();
Example of Fast-Paginate Package Usage
Suppose you have a contacts
table with 200,000 records, and you want to display 15 records per page. You can use the fastPaginate()
method like this:
$contacts = Contact::query()->fastPaginate(15);
Then, you can use the $contacts
variable as usual:
@foreach ($contacts as $contact)
<p>{{ $contact->name }}</p>
@endforeach
{{ $contacts->links() }}
To check the performance of the Fast-Paginate package, you can use the getQueryLog()
method of the DB facade to see the executed SQL statements. For example:
DB::enableQueryLog();
$contacts = Contact::query()->fastPaginate(15);
dd(DB::getQueryLog());
You will see two executed SQL statements: one for the subquery to fetch IDs and one for the main query to fetch the full rows. You can compare their execution time with the regular paginate()
method to observe the difference.
Conclusion
In this article, I introduced the Fast-Paginate package for Laravel, an extension that enhances pagination performance by using a "deferred join" SQL method. I also provided instructions on installing and using this package, along with an illustrative example. I hope this article helps you understand and apply this package in your Laravel projects. If you have any questions or suggestions, please feel free to leave a comment below. Thank you for reading!