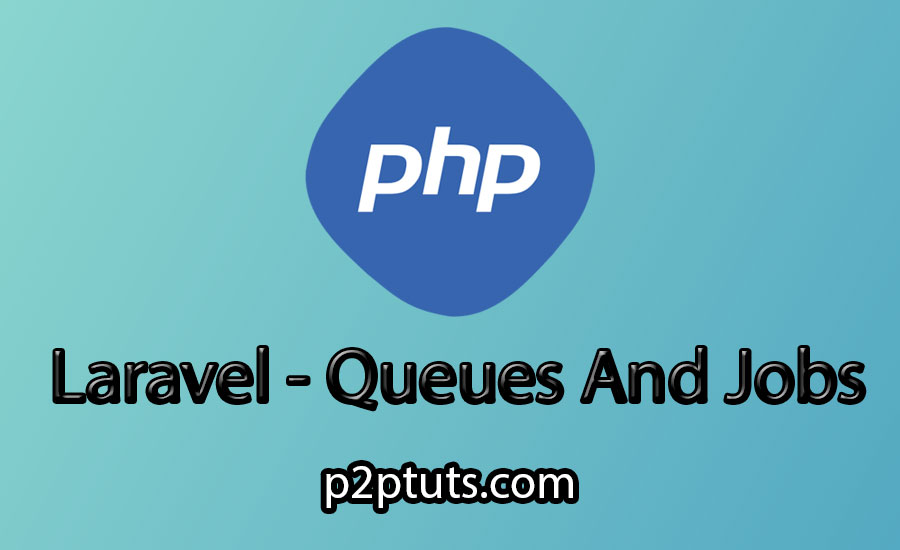
Laravel - Queues And Jobs
Laravel queues and jobs are pivotal concepts for handling complex tasks. Queues organize and execute jobs without delaying the main application. Each job, representing a specific task, can run asynchronously, enhancing performance and ensuring a smooth user experience. Laravel supports multiple queue drivers such as Redis, Beanstalkd, or Amazon SQS, providing flexibility for queue storage and processing. With queues and jobs, Laravel addresses both complex tasks and ensures high performance in the application development environment
Laravel Queue: Unveiling its Essence
Queueing is all about arranging things in a sequential order. Imagine a queuing system in a store that manages customer service based on a first-come, first-served basis.
This concept aligns with Laravel Queue. It performs similar tasks by ensuring services are executed in a specific order.
In the following sections, I'll walk you through a straightforward example to deepen your understanding of Laravel Queue.
Configuring Laravel Queue
To begin with, execute the following commands to create a 'jobs' table that will contain the tasks to be executed.
php artisan queue:table
php artisan migrate
Next, employ the database as the queue driver by updating the .env file accordingly.
QUEUE_CONNECTION=database
Subsequently, create a job with the responsibility of adding dummy data to the 'users' table. To achieve this, run the following command:
php artisan make:job CreateJob
Afterwards, open the CreateJob.php file located in the app/Jobs directory and make the necessary edits:
<?php
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldBeUnique;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
class CreateJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
/**
* Create a new job instance.
*
* @return void
*/
public function __construct()
{
//
}
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
\App\Models\User::factory(10)->create();
}
}
Verifying Laravel Queue Operation
In this step, add a controller to execute the job. Execute the following command to accomplish this:
php artisan make:controller TestQueueJobController
Next, open the TestQueueJobController and make the required adjustments:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Jobs\CreateJob;
class TestQueueJobController extends Controller
{
/**
* Test queue job
**/
public function run()
{
$createUserJob = new CreateJob();
$this->dispatch($createUserJob);
}
}
Then, open routes/web.php and add the line below:
Route::get('test', [App\Http\Controllers\TestQueueJobController::class,'run']);
Afterwards, open your browser and visit the /test-queue-job URL to execute the user addition task.
Upon completion of a job, handle the queue using the following command:
php artisan queue:work
Finally, after the task is completed, you will have ten new users added to the 'users' table.
See more: PHP json_decode()
Handling Queue Closure Errors
In cases where a job fails, you might want to send notifications to your users. To achieve this, add the 'failed' method to your job class.
/**
* The job failed to process.
*
* @param Exception $exception
* @return void
*/
public function failed(\Throwable $exception)
{
}
Customizing Retry Time After Job Failure
Introduce the 'backoff()' method to your Job class, returning an array of integers to specify the waiting time between retries if unsuccessful.
/**
* Calculate the number of seconds to wait before retrying the job.
*
* @return array
*/
public function backoff()
{
return [1, 5, 10];
}
For instance, if the job fails on the first attempt, it will wait for 1 second before retrying. Subsequently, if it continues to fail on the second attempt, it will wait for 5 seconds before retrying. After that, if the job persists in failing on the third attempt and subsequent attempts, it will wait for 10 seconds before retrying.
To ensure a job is executed once or more, add the $tries property to your Job class.
/**
* The number of times the job may be attempted.
*
* @var int
*/
public $tries = 3;
Additionally, you can limit the maximum number of runs for a Job by adding the $maxExceptions property to your Job class.
/**
* The maximum number of unhandled exceptions to allow before failing.
*
* @var int
*/
public $maxExceptions = 3;
You can also specify the maximum number of seconds a Job is allowed to run by adding the $timeout property to your Job class.
/**
* The number of seconds the job can run before timing out.
*
* @var int
*/
public $timeout = 120;
To identify which Job didn't complete within the specified time, simply add the $failOnTimeout property with a value of true to your Job class.
Ignoring Missing Models
If data within a model is deleted while a Job is in the queue, the job will fail. To handle this conveniently, automatically remove the Job when there's no model data by adding the $deleteWhenMissingModels property with a value of true.
/**
* Delete the job if its models no longer exist.
*
* @var bool
*/
public $deleteWhenMissingModels = true;
With these steps, you've completed a simple example of Laravel Queue. I hope this guide proves beneficial in your work. Should you have any questions, feel free to contact us through the contact page. Thank you.