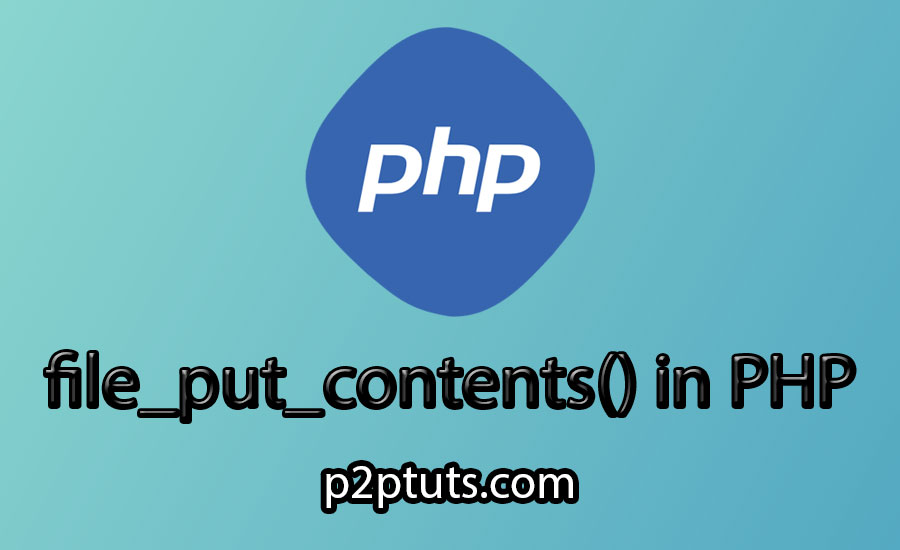
PHP file_put_contents
I. Introduction
PHP, a powerful programming language, provides numerous functions to manipulate files, with php file_put_contents
being a crucial tool for flexible data writing. This article provides an in-depth look at the syntax, parameters, and practical examples of using this function.
II. Syntax and Parameters of file_put_contents
The syntax of file_put_contents
is as follows:
file_put_contents($filename, $data, $flags, $context);
$filename
: The name of the file to write data into.$data
: The content to be written into the file.$flags
: Control flags for data writing, such asFILE_APPEND
to append data at the end of the file.
FILE_USE_INCLUDE_PATH
option: the function will search for$filename
in the include directory; refer to theinclude_path
for further understanding.FILE_APPEND
: if the file already exists, the function will not overwrite the existing content with$data
, but will append$data
to the end of the file.LOCK_EX
: Obtains an exclusive lock on the file during the writing process. In other words, aflock()
call occurs between thefopen()
andfwrite()
calls. This is different from afopen()
call with the "x" mode.
$context
: An optional context used with data from thestream_context_create()
.
III. Examples of Using file_put_contents
1. Save a string to a file
$content = "Content ";
$file = "example.txt";
file_put_contents($file, $content);
2. Save an array to a file
$data = array("A", "B", "C");
$file = "abc.txt";
file_put_contents($file, implode("\n", $data));
3. Save the content of a variable to a file
$variableData = "Data from a variable";
$file = "variable_data.txt";
file_put_contents($file, $variableData);
4. Append content to the end of a file
$newContent = "New content ";
$file = "example.txt";
file_put_contents($file, $newContent, FILE_APPEND);
IV. Handling Errors when using file_put_contents
When using file_put_contents
, it is crucial to check the result of data writing to handle errors effectively:
$file = "example.txt";
$content = "Test content.";
if (file_put_contents($file, $content) !== false) {
echo "Data written successfully!"; }
else {
echo "Failed to write data!";
}
V. Related Functions to file_put_contents
Apart from file_put_contents
, several functions such as file_get_contents
, fopen
, and fwrite
also support file operations in PHP.
VI. Real-world Use Cases of file_put_contents
file_put_contents
is often preferred when quick data writing to files is required, such as saving configurations, logging, or creating cache files. Its simplicity and efficiency make it a popular choice in PHP applications.
In conclusion, file_put_contents
is a vital tool for convenient file data management in PHP. Understanding its syntax and parameters, combined with flexible application in real-world scenarios, helps optimize the performance and stability of PHP applications.