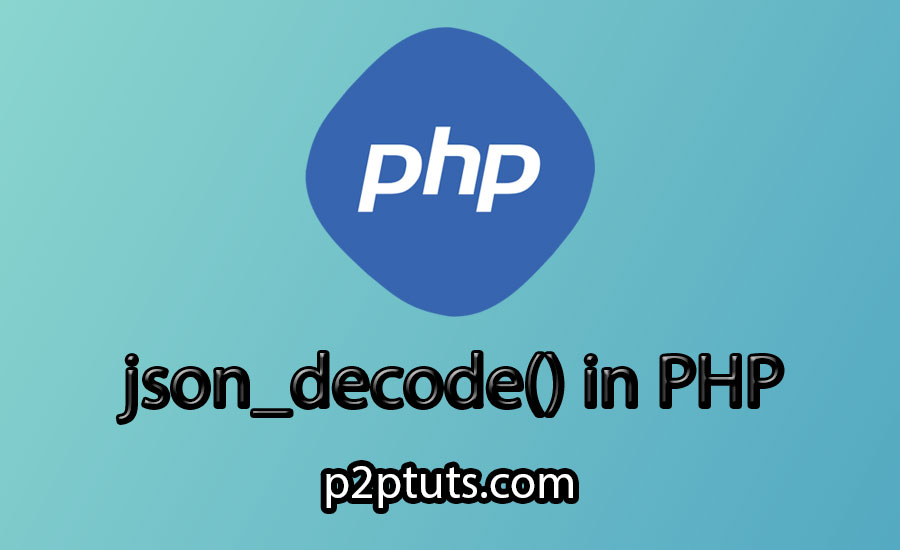
PHP json_decode()
Introduction to the json_decode() Function in PHP
In PHP, json_decode()
plays a crucial role in extending JSON processing capabilities, converting JSON strings into PHP data structures. It serves as a key tool when interacting with data from web APIs, handling JSON storage, and parsing data structures.
Syntax and Parameters of the json_decode() Function
The detailed syntax of the function is as follows:
mixed json_decode(string $json [, bool $assoc = false [, int $depth = 512 [, int $options = 0 ]]])
$json
: The JSON string to be decoded.$assoc
: Iftrue
, the returned result will be an associative array instead of an object.$depth
: The maximum recursion depth specified by the user.$options
: Bitmask of JSON decode options.
Illustrative Examples of Using the json_decode() Function
Various Decoding Options in the json_decode() Function
The json_decode()
function supports multiple options to adjust the JSON decoding process:
// Using JSON_BIGINT_AS_STRING to keep large numbers as strings
$json_bigint = '{"number": 12345678901234567890}';
$decoded_bigint = json_decode($json_bigint, false, 512, JSON_BIGINT_AS_STRING);
Possible Exceptions and Errors When Using json_decode()
During the use of json_decode()
, exceptions and errors can occur, especially when the JSON string is invalid or the recursion depth exceeds the limit.
// Handling errors using JSON_THROW_ON_ERROR
$json_error = '{"name": "Invalid JSON}';
try {
$decoded_error = json_decode($json_error, true, 512, JSON_THROW_ON_ERROR);
} catch (JsonException $e) {
echo 'Error: ', $e->getMessage();
}
Tips and Tricks When Using json_decode()
- Always check the returned result and handle errors carefully.
- Use
json_decode()
with theJSON_OBJECT_AS_ARRAY
option to ensure the result is an associative array when needed. - Check the recursion depth to avoid resource consumption issues.
Comparison of json_decode() with Other JSON Data Handling Functions in PHP
In PHP, json_decode()
primarily focuses on decoding JSON into PHP data structures. Compared to other functions like json_encode()
or json_last_error()
, it provides flexibility and convenience when handling input data.
Real-World Applications of the json_decode() Function in PHP Programming
- Interacting with web APIs returning JSON data.
- Parsing and handling JSON data from storage sources such as NoSQL databases.
- Reading and understanding data from JSON-formatted configuration files.
Reference Documentation and Useful Information Sources on json_decode()
- Official PHP documentation on json_decode()
- W3Schools - JSON in PHP
Summary and Evaluation of the json_decode() Function in PHP
json_decode()
plays a vital role in processing JSON data in PHP, providing flexible and efficient decoding capabilities. This makes it a powerful tool for interacting with JSON data sources and seamlessly integrating them into PHP applications.