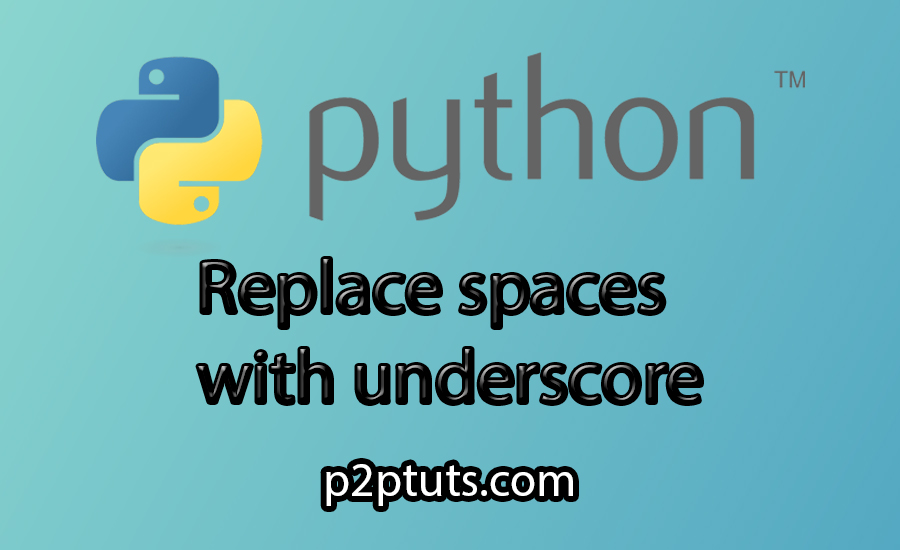
How to replace whitespace with underscore in python
Python is a popular and versatile programming language with many features and useful libraries. In this article, we will explore different ways to python replace spaces with underscores (_) in Python.
Syntax for replace() method
A simple and convenient way to replace spaces with underscores in Python is to use the replace()
method of the str
class. This method takes two parameters: the string to be replaced and the replacement string. It returns a new string after performing the replacement.
Example:
s = "P2P Tuts"
s = s.replace(" ", "_")
print(s)
Output:
P2P_Tuts
split() and join()
Another way to replace spaces with underscores in Python is to use the split()
and join()
methods of the str
class. The split()
method separates a string into a list of substrings based on a delimiter. The join()
method concatenates a list of strings into a single string using a specified delimiter.
Example:
s = "P2P Tuts"
s = "_".join(s.split(" "))
print(s)
Output:
P2P_Tuts
String using for loop
Another approach to replace spaces with underscores in Python is to use a for
loop to iterate through each character in the string. Check if the character is a space, then replace it with an underscore. After that, concatenate the results into a new string.
Example:
s = "P2P Tuts"
result = ""
for c in s:
if c == " ":
result += "_"
else:
result += c
print(result)
Output:
P2P_Tuts
String using Regex sub()
Another method to replace spaces with underscores in Python is to use a regular expression (regex) to search and replace spaces in the string. To do this, we need to import the re
library and use its sub()
method. This method takes three parameters: the regex pattern, the replacement string, and the input string. It returns a new string after performing the replacement.
Example:
import re
s = "P2P Tuts"
s = re.sub("\s", "_", s)
print(s)
Output:
P2P_Tuts
Summary
In this article, we learned how to replace spaces in a string with underscores (_) in different ways in Python. These methods include:
- Using the
replace()
method of thestr
class - Using the
split()
andjoin()
methods of thestr
class - Using a
for
loop to iterate through each character in the string - Using a regular expression (regex) to search and replace spaces in the string
P2P Tuts I hope this article is helpful for you in learning and programming in Python. If you have any questions or suggestions, please feel free to leave a comment below. Thank you for reading! 😊.