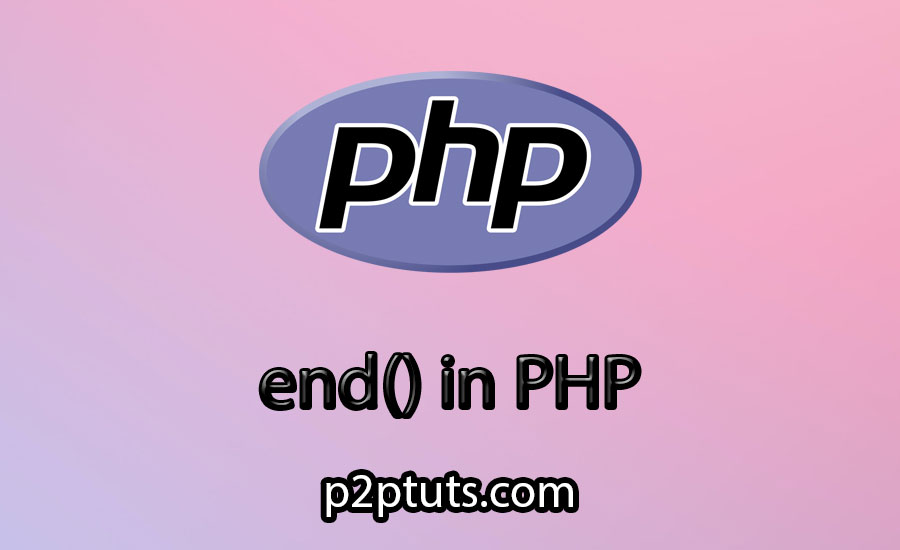
Function end() in PHP
- by Admin
- 163 Views
- PHP
- Codeigniter
- Laravel
In PHP programming, the end()
function serves as a crucial tool for flexible array manipulation, especially when there is a need to access and work with the last element of an array. This article provides a detailed overview of the end()
function and how to use it effectively.
Syntax of the end()
Function in PHP
The basic syntax of the end()
function is as follows:
mixed end(array &$array)
&$array
: The array to be processed, and the use of&
signifies referencing the original array, allowing the function to modify the original array if necessary.
How to Use the end()
Function in PHP
The end()
function is designed to move the array pointer to the last element, making it easy to access and perform operations on the last value of the array.
This function has only one main parameter, which is the array to be processed, passed by reference to allow modification of the original array.
The end()
function returns the last value of the array after the pointer has been moved.
Illustrative Examples of Using the end()
Function in PHP
<?php
$numbers = array(1, 2, 3, 4, 5);
// Move the pointer to the last element of the array
end($numbers);
// Get the last value
$lastValue = current($numbers);
echo "The last value of the array is: $lastValue"; // 5
?>
The end()
function is often applied when extracting the last value of an array, especially in situations where the size of the array is not known beforehand.
When using the end()
function, it is essential to ensure that the array is not empty to avoid unexpected errors when the pointer cannot be moved to any element.
There are several related functions, such as reset()
to reset the pointer to the beginning of the array and prev()
to move the pointer to the previous element.
Reference Documentation
For more in-depth information and richer examples regarding the end()
function and related functions, you can refer to the official PHP documentation on the php.net website.
Keywords:
- Get last item in array PHP
- Array function PHP
- Get first element of array PHP
- Check last key in foreach php
- Push object to array PHP
- Array pop PHP
- Str split PHP
- Each PHP