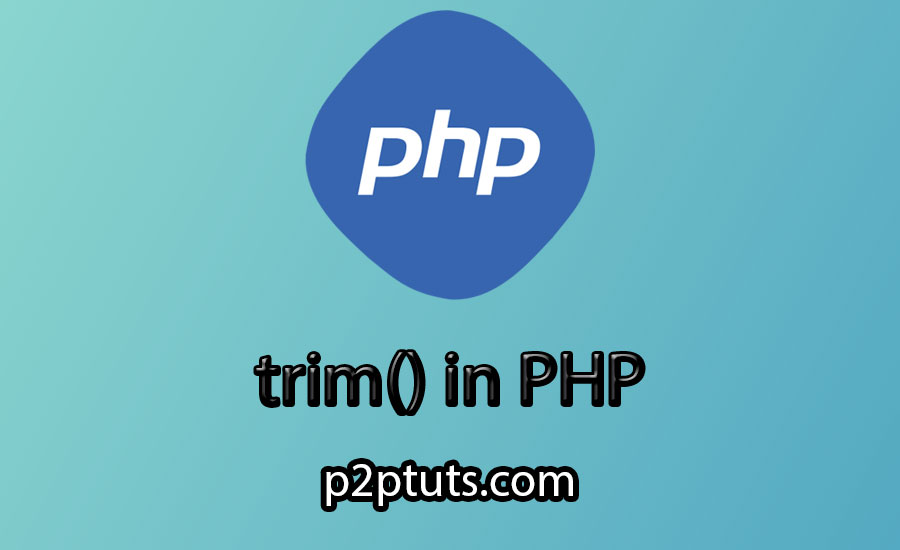
Instructions for using the trim() function in PHP
- by Admin
- 206 Views
- PHP
- Codeigniter
- Laravel
Introduction
The trim()
function in PHP is a powerful string manipulation tool designed to eliminate leading and trailing whitespace or specified redundant characters from a string. Its purpose is to refine strings by removing unnecessary spaces or characters, ensuring data accuracy and uniformity.
Syntax
The trim()
function is structured as follows:
trim($str, $charlist)
Where:
$str
represents the string from which characters are to be removed at the beginning and end.$charlist
is an optional parameter specifying the characters to be removed from the string's start and end. If omitted,trim()
defaults to removing characters such as\0
(NULL),\t
(tab),\n
(new line),\x0B
(vertical tab),\r
(carriage return), and (white space).
Arguments of the trim()
function in PHP
The arguments for trim()
in PHP can be a single character or a list of characters. For instance:
- If
$charlist
is a single character, like"M"
,trim()
removes all occurrences of"M"
at the string's beginning and end. - If
$charlist
is a list, e.g.,"MTL"
,trim()
removes"M"
,"T"
,"L"
characters from both ends of the$str
string, respecting their order of appearance¹².
Return value of the trim()
function in PHP
The trim()
function returns a new string with the specified characters removed from both the beginning and end. Importantly, this operation does not alter the original string.
Examples of using the trim()
function in PHP
Here are some examples illustrating the use of the trim()
function in PHP:
// Example 1: Remove white spaces at the beginning and end of the string
$str = " Hello World ";
echo trim($str); // Displays: "Hello World"
// Example 2: Remove "M" characters at the beginning and end of the string
$str = "MMMDocumentation for learning HTMLMMMM";
echo trim($str, "M"); // Displays: "Documentation for learning HTML"
Common use cases of the trim()
function in PHP
The trim()
function in PHP finds application in various scenarios, with common use cases including:
- Processing user-input data to eliminate excess spaces or unwanted special characters³.
- Ensuring consistent data for storage and display by comparing two strings, mitigating differences due to leading and trailing characters⁴.
- Normalizing data before storage or presentation to enhance uniformity and ease of manipulation⁵.
Notes when using the trim()
function in PHP
When utilizing the trim()
function in PHP, several key considerations include:
trim()
only removes characters from the beginning and end of the string, leaving characters in the middle unaffected¹.- There's no case sensitivity in
trim()
, meaning it treats uppercase and lowercase characters the same². - Characters with ASCII values exceeding 127, such as Vietnamese characters, are not removed by
trim()
⁶. trim()
cannot delete characters based on a pattern or regular expression. For such cases,preg_replace()
function may be used⁷.
Comparison of trim()
with other functions in PHP
PHP provides two similar functions, ltrim()
and rtrim()
, in addition to trim()
. The distinctions are as follows:
ltrim()
only removes characters from the beginning of the string.rtrim()
exclusively removes characters from the end of the string.trim()
removes characters from both the beginning and end¹.
Example:
$str = " Hello World ";
echo ltrim($str); // Displays: "Hello World "
echo rtrim($str); // Displays: " Hello World"
echo trim($str); // Displays: "Hello World"
Other functions related to string manipulation in PHP
PHP offers numerous functions for string manipulation. Some commonly used ones include:
strlen()
: Retrieve the length of a string.strpos()
: Find the position of the first occurrence of a substring in a string.substr()
: Obtain a portion of a string.str_replace()
: Replace a substring with another string in a string.strtolower()
andstrtoupper()
: Convert a string to lowercase or uppercase.explode()
andimplode()
: Split or join strings using a delimiter.
Conclusion
The trim()
function in PHP serves as a valuable string manipulation tool, effectively removing whitespace or specified redundant characters from both ends of a string. Its versatility makes it applicable in various scenarios, from processing user-input data to ensuring consistent data for storage and display.