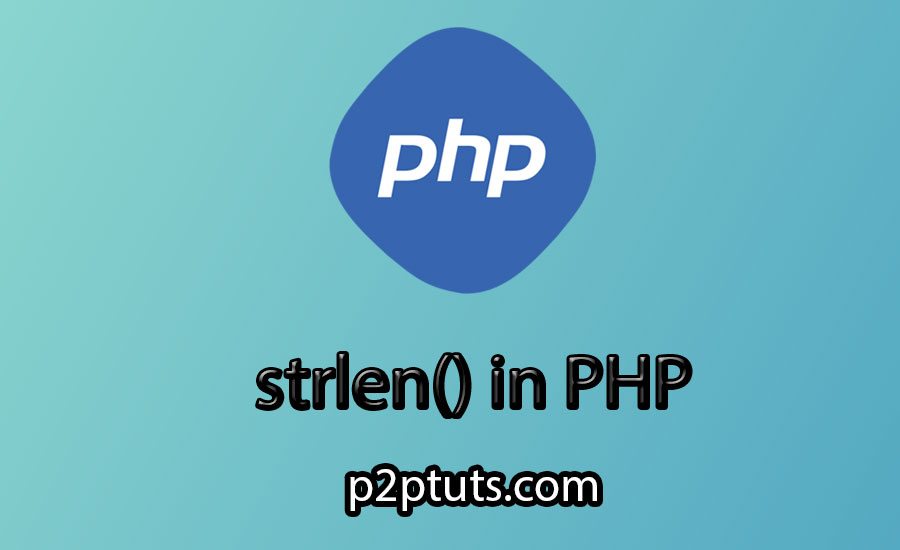
PHP strlen() - Check string length in PHP
- by Admin
- 258 Views
- PHP
- Codeigniter
- Laravel
What is strlen?
PHP strlen
is an essential function used to measure the length of a string. Its primary purpose is to return the number of characters in a string, aiding in checking the size or length of textual data.
Syntax of strlen in PHP
The syntax of strlen
is straightforward:
$length = strlen($str);
Here, $str
is the string whose length needs to be measured, and the value of $length
is the number of characters in the string.
Parameters of strlen
The strlen
function only accepts one parameter, which is the string to measure its length.
Return Value of strlen
The strlen
function returns an integer, representing the length of the input string, counting both special characters and spaces.
Example of strlen
$text = "Hello, World!";
$length = strlen($text);
echo "The length of the string is: " . $length;
The result will be: "The length of the string is: 13".
Some Notes When Using strlen
strlen
does not recognize multi-byte characters in the string.- If the string contains multi-byte characters such as UTF-8, the
mb_strlen()
function can be used as an alternative to ensure accuracy in measuring the string's length.
Extended Examples of strlen
$string1 = "I love PHP!";
$length1 = strlen($string1); // 11
$string2 = "Café au lait";
$length2 = strlen($string2); // 13
$string3 = "مرحبا بك في PHP";
$length3 = strlen($string3); // 19
strlen() vs count_chars()
The difference between strlen
and count_chars()
lies in how they count characters. strlen()
counts all characters in the string, including spaces, whereas count_chars
counts only non-repeating characters.
strlen() vs mb_strlen()
While strlen()
does not recognize multi-byte characters, mb_strlen()
is capable of handling languages with special and multi-byte characters, such as Japanese or Chinese.
Conclusion
The strlen()
function in PHP is a vital tool for checking the length of a string. However, when dealing with languages containing special characters, using mb_strlen()
may be a preferable choice to ensure accuracy in measuring the string's length.