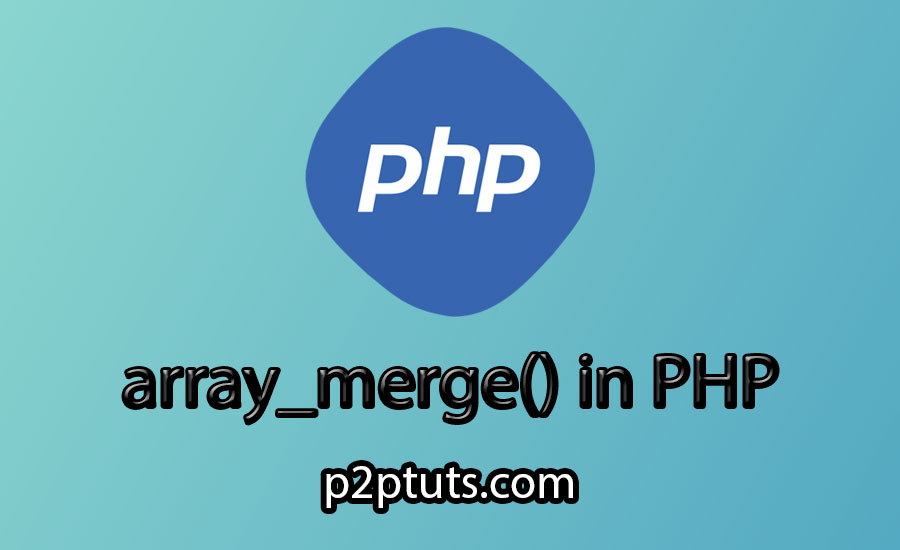
array_merge() - Append To Array PHP
The PHP function array_merge() is a powerful tool for efficiently appending to arrays in PHP. It seamlessly combines multiple arrays into a single array, making it particularly useful for operations like append to array php. Although commonly used for appending associative arrays, it also works seamlessly with sequentially indexed arrays
Syntax:
array_merge(array $array1, array $array2, ...): array
Parameters
$array1, $array2, ...
: Arrays to be merged, and multiple arrays can be passed.
Return Value: A new array containing all elements from the input arrays.
Example:
$array1 = ['fruit' => 'apple', 'color' => 'red'];
$array2 = ['fruit' => 'banana', 'color' => 'yellow'];
$result = array_merge($array1, $array2);
print_r($result);
Output:
Array ( [fruit] => banana [color] => yellow )
Comparison with Other Functions
array_merge()
combines arrays in the order provided without considering keys.array_merge_recursive()
performs recursive merging, whilearray_merge()
overwrites values if there are overlapping keys.
Advantages
- Easy to use with a simple syntax.
- Flexible when dealing with multiple input parameters.
Disadvantages
- Does not intelligently handle overlapping keys like
array_merge_recursive()
.
Real-world Applications
- Creating consolidated data structures from various sources.
- Facilitating the creation of a new array from existing arrays for utility operations.
3 ways to combine 2 arrays in PHP
-
Leveraging array_merge
A widely adopted approach involves employing the array_merge() function. For instance:
$a = array('a', 'b');
$b = array('c', 'd');
print_r(array_merge($a, $b));
Result:
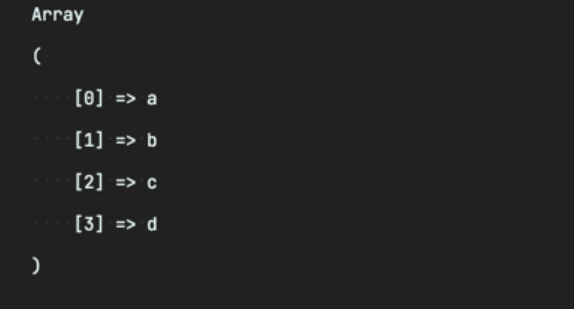
-
Utilizing array_push
Example: Updating both the $b and $a arrays.
$a = array('a', 'b');
$b = array('c', 'd');
array_push($a, ...$b);
print_r($a);
-
Employing a loop
As a final, albeit more manual method, we can use a loop
$fruits = ['Apple','Orange','Mango'];
$more_fruits = ['Strawberry', 'Watermelon','Pear'];
foreach($more_fruits as $fruit)
{
$fruits[] = $fruit;
}
// Output the array to the console.
print_r($fruits);
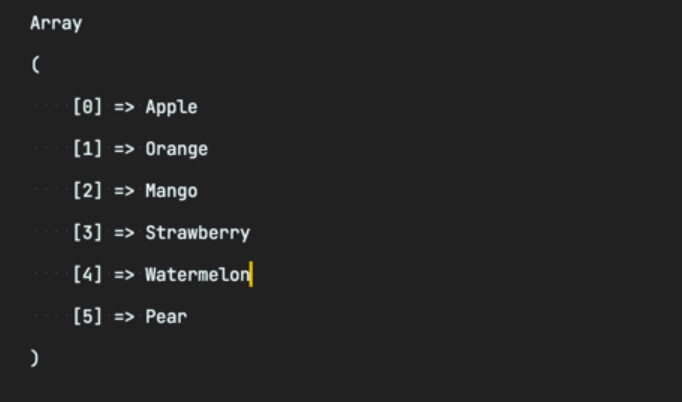
In summary, array_merge() is an extremely useful function in PHP used to merge multiple arrays into a single array. This function can be employed to address a variety of common programming challenges, such as combining data from different sources or creating a new array from elements of various arrays. array_merge is also highly versatile, allowing you to customize the result in many different ways, such as altering the order of elements or eliminating duplicate elements.
- PHP array merge unique
- Array_push
- Array_merge_recursive
- php array_merge duplicate keys
- Array_combine
- PHP merge 2 arrays with same keys
- Array_merge trong PHP
- php array merge