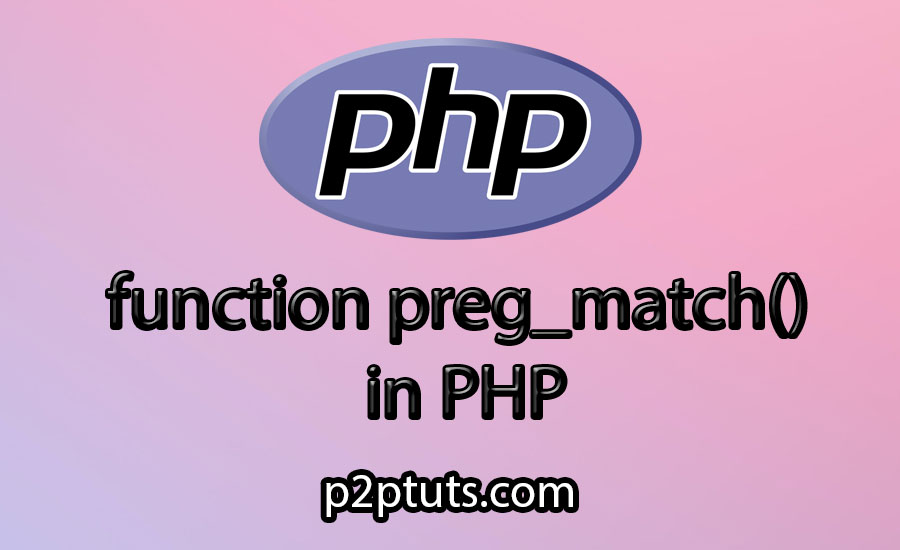
Preg_match function in PHP: detailed instructions for use
- by Admin
- 173 Views
- PHP
- Codeigniter
- Laravel
Introduction
PHP programming is becoming increasingly popular and powerful in web application development. In this development environment, the preg_match function serves as a crucial tool for flexible string data processing and validation. This article delves into the functionality, syntax, parameters, return values, and applications of the preg_match function in PHP.
Syntax
The preg_match function is designed to use regular expressions to match strings. The basic syntax of the function is as follows:
preg_match($pattern, $subject, $matches);
- $pattern: The regular expression you want to check.
- $subject: The string to check against the regular expression.
- $matches: The result array that stores information about the matching results.
Parameters
- Regular expression ($pattern): This is one of the most crucial parameters, determining the matching rules.
- String to be checked ($subject): The place where you want to apply the regular expression for validation.
- Result array ($matches): The variable where the function will store the results of the match.
Return Value
The preg_match function returns a boolean value:
- Returns 1 if at least one match is found.
- Returns 0 if no matches are found.
- Returns FALSE if an error occurs.
Examples
- Checking email format:
$email = "[email protected]";
if (preg_match("/^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/", $email)) {
echo "Valid email!";
} else {
echo "Invalid email!";
}
- Finding words starting with "open" in a text:
$text = "Google is an open research organization.";
if (preg_match("/\bopen\w*/i", $text, $matches)) {
echo "Found word: " . $matches[0];
} else {
echo "No words starting with 'open' found.";
}
VI. Commonly Used Regular Expression Patterns
- Checking phone number:
$phone_number = "123-456-7890";
$pattern = "/^\d{3}-\d{3}-\d{4}$/";
- Finding URLs in text:
$text = "Visit our website at http://www.example.com";
$pattern = "/https?:\/\/[a-zA-Z0-9.-]+\/[a-zA-Z0-9.-]+/";
Tips for Effective Use
- Optimize regular expressions to avoid performance issues.
- Use '^' and '$' to specify the start and end of the string.
Notes
- When using '/', escape them with '' to avoid conflicts with characters in the regular expression.
- If checking multiple conditions, use more complex regular expressions.
Functions Related to preg_match in PHP
- preg_match_all(): Finds all matching results in a string.
- preg_replace(): Replaces matching results with another string.
The preg_match function is widely used for data validation, user input validation, string format checking, and information extraction in web applications. Specifically, it can be applied in form processing systems, data input verification, and information filtering from various data sources.
In conclusion, with a solid understanding of preg_match, PHP developers can leverage its flexibility to build powerful and secure web applications.