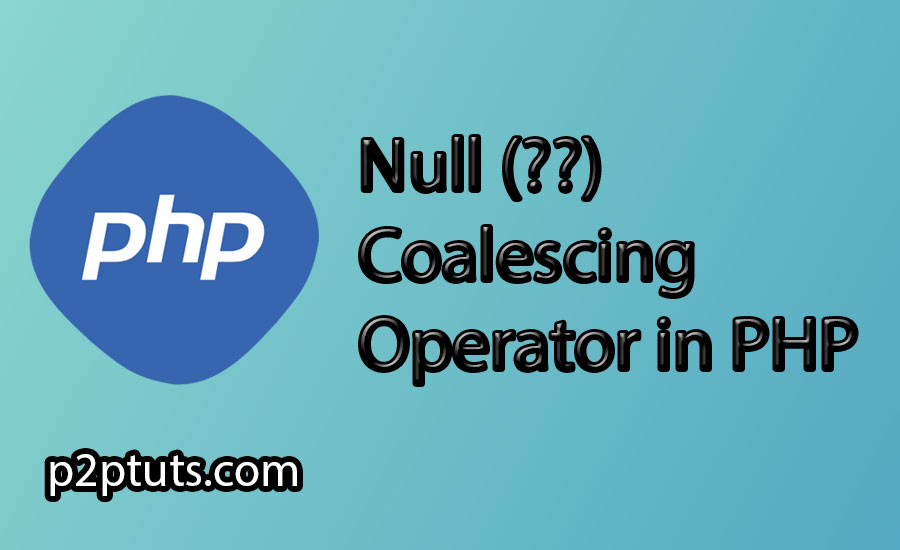
Null Coalescing Operator in PHP: Simplifying Null Value Handling
In the realm of PHP programming, the Null Coalescing operator (??
) serves as a powerful tool to address common challenges associated with handling null values. Introduced since PHP 7, this operator provides concise and readable syntax for efficiently checking and managing null values.
Basic Syntax
$variable = $value ?? $default;
Where:
$variable
: Variable that will take the value if$value
is notnull
.$value
: The value we want to check.$default
: Default value to be used if$value
isnull
.
Exemplary Use Case
$welcomeMessage = $userGreeting ?? "Hello, Guest!";
echo $welcomeMessage;
If $userGreeting
is not null
, then $welcomeMessage
will take the value of $userGreeting
. Otherwise, if $userGreeting
is null
, $welcomeMessage
will default to "Hello, Guest!".
Advantages of the Null Coalescing Operator:
-
Concise Source Code: Enables writing more concise code compared to traditional checks like
isset()
or the ternary (? :
) operator. -
Flexible Null Value Handling: Simplifies the process of handling null values, avoiding unintended errors in the source code.
Examples:
1. Managing User Color Preferences
$userColorPreference = $userSettings['color'] ?? "default";
echo "User's preferred color: " . $userColorPreference;
If $userSettings['color']
is not null
, $userColorPreference
will take that value. If $userSettings['color']
is null
, the default value "default" will be used.
2. Displaying User Age Information
$userProfile = ['name' => 'Alice', 'age' => null, 'email' => '[email protected]'];
$userAge = $userProfile['age'] ?? 'N/A';
echo "User's age: " . ($userAge !== null ? $userAge : 'Not Available');
In the $userProfile
array, if the value of 'age'
is not null
, the variable $userAge
will take that value. If 'age'
is null
, the default value 'N/A' will be used.
3. Welcoming Users with a Special Name
$userName = $userDetails['name'] ?? 'Guest';
echo "Welcome, " . htmlspecialchars($userName, ENT_QUOTES, 'UTF-8') . "!";
If $userDetails['name']
is not null
, $userName
will take that value. If $userDetails['name']
is null
, the default value 'Guest' will be used.
PHP ?? operator These examples vividly illustrate how the Null Coalescing operator streamlines source code and enhances richness when dealing with null values in PHP.
Keywords:
- PHP
- Ternary operator PHP
- PHP online
- PHP bitwise operators
- PHP null coalescing operator
- Spread operator PHP
- Empty coalescing operator php
- Assign php